A path in a binary tree is a sequence of nodes where each pair of adjacent nodes in the sequence has an edge connecting them. A node can only appear in the sequence at most once. Note that the path does not need to pass through the root.
The path sum of a path is the sum of the node's values in the path.
Given the
Example 1:
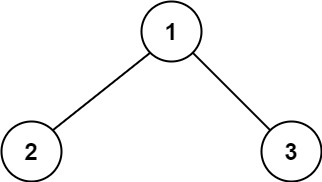
Input: root = [1,2,3] Output: 6 Explanation: The optimal path is 2 -> 1 -> 3 with a path sum of 2 + 1 + 3 = 6.
Example 2:
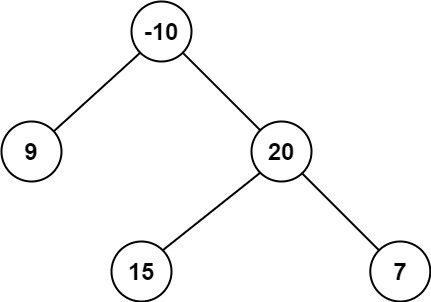
Input: root = [-10,9,20,null,null,15,7] Output: 42 Explanation: The optimal path is 15 -> 20 -> 7 with a path sum of 15 + 20 + 7 = 42.
Constraints:
- The number of nodes in the tree is in the range
[1, 3 * 104] . -1000 <= Node.val <= 1000
歷遍求極值,然後資料結構是tree,應該會是用 DFS 來解
這題麻煩的應該是條件的限制,因為最大值的 path,不一定會發生在 root 的地方
也可以想成,每個 node 都可以是最大值發生的 root node
所以在 DFS 的過程中,一直去紀錄最大值是多少
然後有個要注意的是,每個 DFS 的回傳值,必須從 left 和 right 之中選一個
原因是題目規定,同一個 path 只能出現一次
如果 left 和 right 都選了,則他們兩個人的 parent node 就會出現兩次,不符合規定
Kotlin
沒有留言:
張貼留言