You are given two lists of closed intervals,
Return the intersection of these two interval lists.
A closed interval
The intersection of two closed intervals is a set of real numbers that are either empty or represented as a closed interval. For example, the intersection of
Example 1:
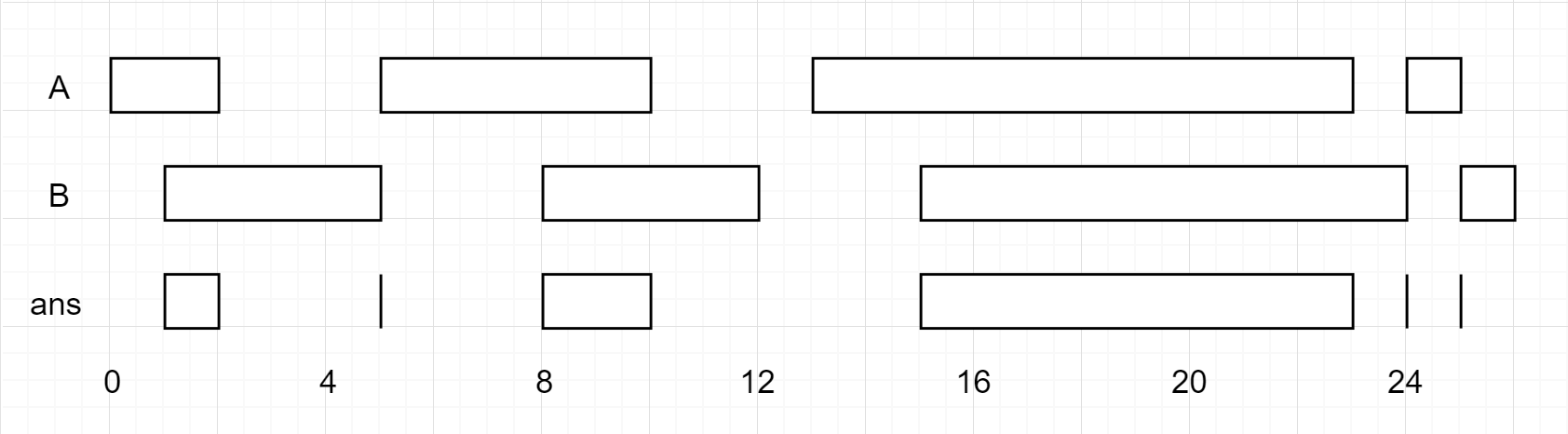
Input: firstList = [[0,2],[5,10],[13,23],[24,25]], secondList = [[1,5],[8,12],[15,24],[25,26]] Output: [[1,2],[5,5],[8,10],[15,23],[24,24],[25,25]]
Example 2:
Input: firstList = [[1,3],[5,9]], secondList = [] Output: []
Example 3:
Input: firstList = [], secondList = [[4,8],[10,12]] Output: []
Example 4:
Input: firstList = [[1,7]], secondList = [[3,10]] Output: [[3,7]]
Constraints:
0 <= firstList.length, secondList.length <= 1000 firstList.length + secondList.length >= 1 0 <= starti < endi <= 109 endi < starti+1 0 <= startj < endj <= 109 endj < startj+1
Solution
這個是要取兩個list中交集的部分,且這兩個 list 自己裡面的區間沒有重疊,且是排過的
想法如下
因為是取交集,start 會是兩者間比較大的,end 會是兩者之間比較小的
且還要確認 start <= end,才是合法的交集區間
如果有找到,就存到 ans 裡面
那接下是要怎麼移動,檢查當下誰的結束時間比較晚
晚的那個,表示還有機會和其他區間有交集
所以晚的那個不動,移動結束時間比較早的那個
kotlin
沒有留言:
張貼留言